Integration Field
Proposales uses a simple JSON based schema for defining UI layouts. These allow the user to customize parts of the UI. Currently customization is possible in the following places: integration config, proposal content details, proposal editor widget, and proposal data widget.
Icons
Some of the integration fields can be customized with an icon. The icon is specified by name. Currently the following icons are supported:
Select
The select field creates a select input.

Text Input
The text input can be of various HTML text input types, and can be customized with optional icons.

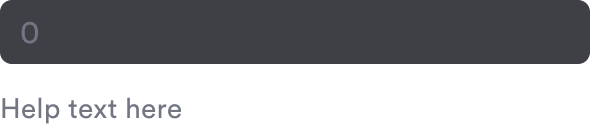
Number Input
This works the same as text fields, but with numeric values.


Hidden field
Hidden fields are useful for setting properties internally without showing them them to the user. For example default values in a integration config. These are not visible in the UI, and are set to the default value specified.
Switch
A toggleable switch for boolean values.

Link button
A button linking to an external page.
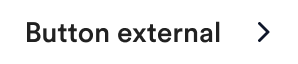
Divider
A horizontal divider with an optional label
Header
A header for dividing fields into sections.
Text
A section of text, with optional markdown support.